Corporate website
Corporate portal for the company!
Internet-shop
Modern and fast website!
Social network
Unique solutions!
Personal website
For your business!
WEB
Full stack Web development...
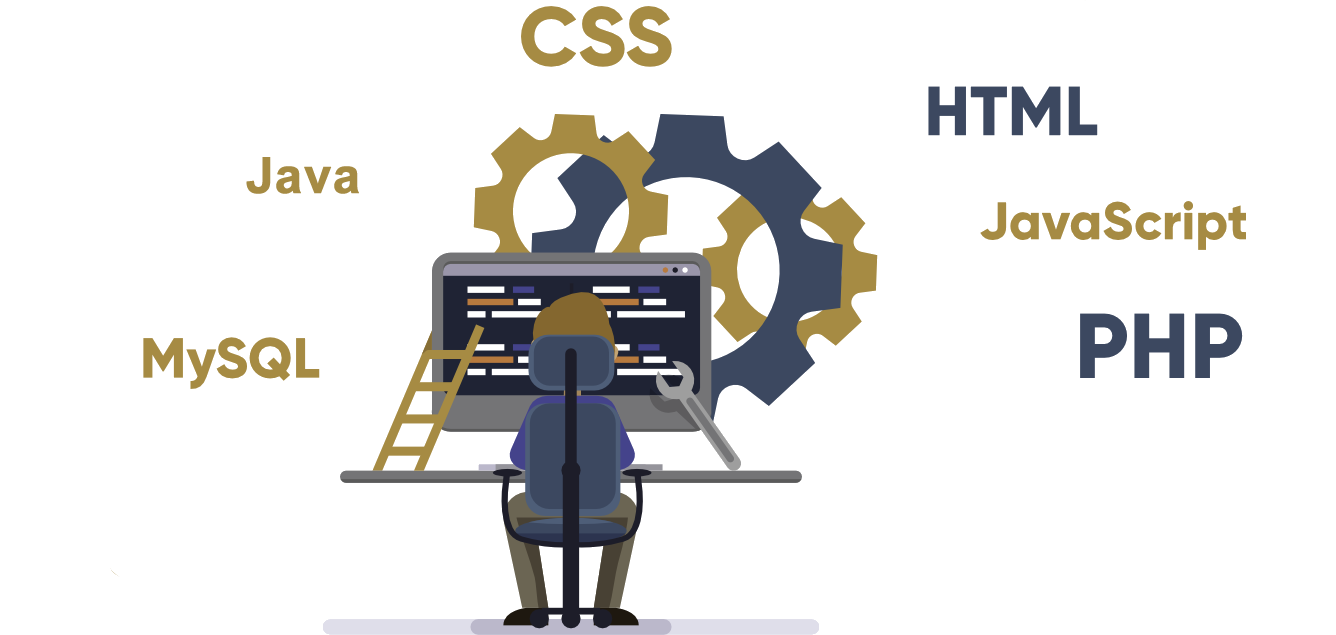
OUR SOLUTIONS PHP PDO MySQL
PHP Data Objects (PDO).
Application of the PDO extension... Code example
PHP PDO is a specialized universal class that implements a database access interface. It provides interaction with databases using objects and allows you to connect to many different types of databases (MySQL, PostgreSQL, SQLite, Oracle, Microsoft SQL Server and others)...
You should always remember to ensure the security of your application, in particular, protection against SQL injections. The application of prepared PDO queries to the MySQL database provides such protection! This is the only safe way to execute SQL queries using variables!
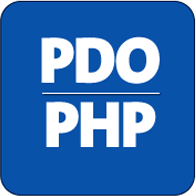
Db.php (Db class - a component for working with a database)
class Db
{
/**
* Establishes a connection to the database
* @return An object of the PDO class for working with a database
*/
public static function getConnection()
{
// Getting connection parameters from a file
$paramsPath = ROOT . '/config/db_params.php';
$params = include($paramsPath);
// Establishing a connection
$dsn = "mysql:host={$params['host']};dbname={$params['dbname']}";
$db = new PDO($dsn, $params['user'], $params['password']);
// Setting the encoding
$db->exec("set names utf8");
return $db;
}
}
db_params.php (Array with database connection parameters)
return array(
'host' => 'YourHost',
'dbname' => 'YourDbName',
'user' => 'YourUserName',
'password' => 'YourPassword',
);
Example of a prepared database query in the model User.php
/**
* The User class is an example of a model for working with users
*/
class User
{
/**
* User Registration
* @param array $options (Array with user information)
* @return integer (id of the record added to the user table)
*/
public static function register($options)
{
$name = $options['name'];
$email = $options['email'];
$password = $options['password'];
/* !!! All incoming data must be cleared to eliminate the XSS (cross-site scripting) vulnerability !!!
* $name = strip_tags($options['name']);
* $name = htmlentities($options['name'], ENT_QUOTES, "UTF-8");
* $name = htmlspecialchars($options['name'], ENT_QUOTES);
* !!! The password ($password) must be encrypted using a strong encryption algorithm !!!
*/
// Connecting to the database
$db = Db::getConnection();
// The text of the database request
$sql = 'INSERT INTO user (name, email, password)'
. 'VALUES (:name, :email, :password)';
// Receiving and returning results. A prepared query is used.
$result = $db->prepare($sql);
$result->bindParam(':name', $name, PDO::PARAM_STR);
$result->bindParam(':email', $email, PDO::PARAM_STR);
$result->bindParam(':password', $password, PDO::PARAM_STR);
if ($result->execute()) {
// If the request is successful, we return the ID of the record added to the table.
return $db->lastInsertId();
}
// Otherwise we return 0
return 0;
}
}
Here is an example of a generic PHP PDO class and implementation of a database access interface...
Be sure to use a software development methodology that prevents the introduction of vulnerabilities, provides resistance to malware and unauthorized access!