Corporate website
Corporate portal for the company!
Internet-shop
Modern and fast website!
Social network
Unique solutions!
Personal website
For your business!
WEB
Full stack Web development...
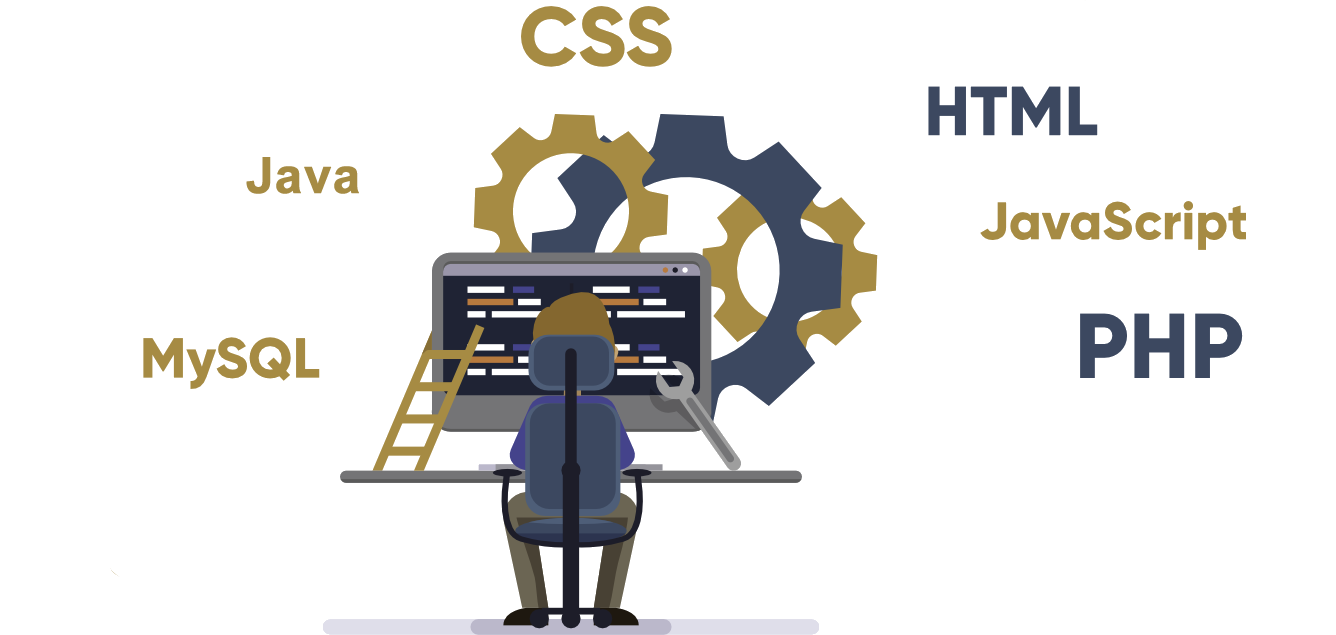
OUR SOLUTIONS JS
HTTP requests (JavaScript).
Using asynchronous XMLHttpRequest... Code example
XMLHttpRequest is a built-in JavaScript object, used to communicate with servers and load the content of web pages without restarting the browser...
AJAX is used to make asynchronous HTTP requests. This means that while waiting for a response, other parts of your code may continue to run... AJAX works with the XMLHttpRequest object by default!
Sending a POST request in JavaScript using XMLHttpRequest
// Once the page loads
window.onload = function () {
// Checking browser support for FormData
if(!window.FormData) {
alert("Your browser does not support downloading files!");
}
}
$(document).ready(function(){
// Define an event when the form submit button is clicked (button id="your-form-send")
const yourFormSend = document.getElementById('your-form-send');
yourFormSend.addEventListener('click', (e) =>{
e.preventDefault();
// Create an XMLHttpRequest object
var request = new XMLHttpRequest();
// Create Request Object
request.open("POST", "Path to the form data handler script");
// Setting the request header
request.setRequestHeader("X-Requested-With", "XMLHttpRequest");
// Defining an event listener for readystatechange
request.onreadystatechange = function() {
// Checking the success of the request
if(this.readyState === 4 && this.status === 200) {
// Insert the response from the server into an HTML element with id="server_response"
document.getElementById("server_response").innerHTML = this.responseText;
}
};
// Retrieving form data id="your-form"
var yourForm = document.getElementById("your-form");
var formData = new FormData(yourForm);
// Send a request to the server
request.send(formData);
// Clearing form fields after receiving a server response
document.getElementById("your-form").reset();
});
})
Asynchronous request in jQuery using $.ajax() method
$(document).ready(function(){
$("#your-form-send").click(function(){
$.ajax({
type: "POST",
url:"Path to the form data handler script",
data:$("#your-form").serialize(),
error:function(){$("#server_response").html("An error has occurred!");},
beforeSend: function(){
$("#server_response").html("Sending data...");
},
success: function(result){
$("#server_response").html(result);
$("#your-form")[0].reset();
}
});
return false;
});
});
Everything is simple, if you don’t complicate anything!
Here are examples of asynchronous POST requests using XMLHttpRequest and the $.ajax() method in jQuery...
Validate all user input on the server side! Be sure to use a software development methodology that prevents the introduction of vulnerabilities, provides resistance to malware and unauthorized access!